the development platform:
1) Python 3.3.5x86-64 installer from https://www.python.org/downloads/release/python-335/
2) PyQt5 64 bit installer from http://www.riverbankcomputing.com/software/pyqt/download5
3) cx_freeze for Python 3.3 64 bit from http://cx-freeze.sourceforge.net/
install those applications, after that open windows command prompt by clicking start -> run (or Winkey+r shortcut)-> cmd -> enter, change directory to your python installion path, 'cd \python33' (change the path if you are not using default path and type without quotes)
then run ' python.exe -c "import sys; print (sys.version)" ' in the prompt, you will get output like this,
3.3.5 (v3.3.5:62cf4e77f785, Mar 9 2014, 10:35:05) [MSC v.1600 64 bit (AMD64)]'
run ' path ', you will get,
PATH=C:\Python33\Lib\site-packages\PyQt5; ... more path here ...
also, check these paths exist, if they doesn't exist, there is something wrong of the installation
C:\Python33\Lib\site-packages\cx_Freeze
C:\Python33\Lib\site-packages\PyQt5
the hello world source code from https://gist.github.com/mizki9577/6856389
C:\Python33\Lib\site-packages\PyQt5
the hello world source code from https://gist.github.com/mizki9577/6856389
[helloqt.py]
#!/usr/bin/env python3
#-*- coding: utf-8 -*-
"""
helloqt.py
PyQt5 で Hello, world!
"""
import sys
from PyQt5 import QtWidgets
def main():
app = QtWidgets.QApplication(sys.argv)
window = QtWidgets.QMainWindow()
button = QtWidgets.QPushButton("Hello, PyQt!")
window.setCentralWidget(button)
window.show()
app.exec_()
if __name__ == '__main__':
main()
copy and paste the code to your IDE, for example, PyCharm, at this moment you can use windows notepad and save the file to (for example): C:\Users\Administrator\Desktop\py33src\helloqt.py
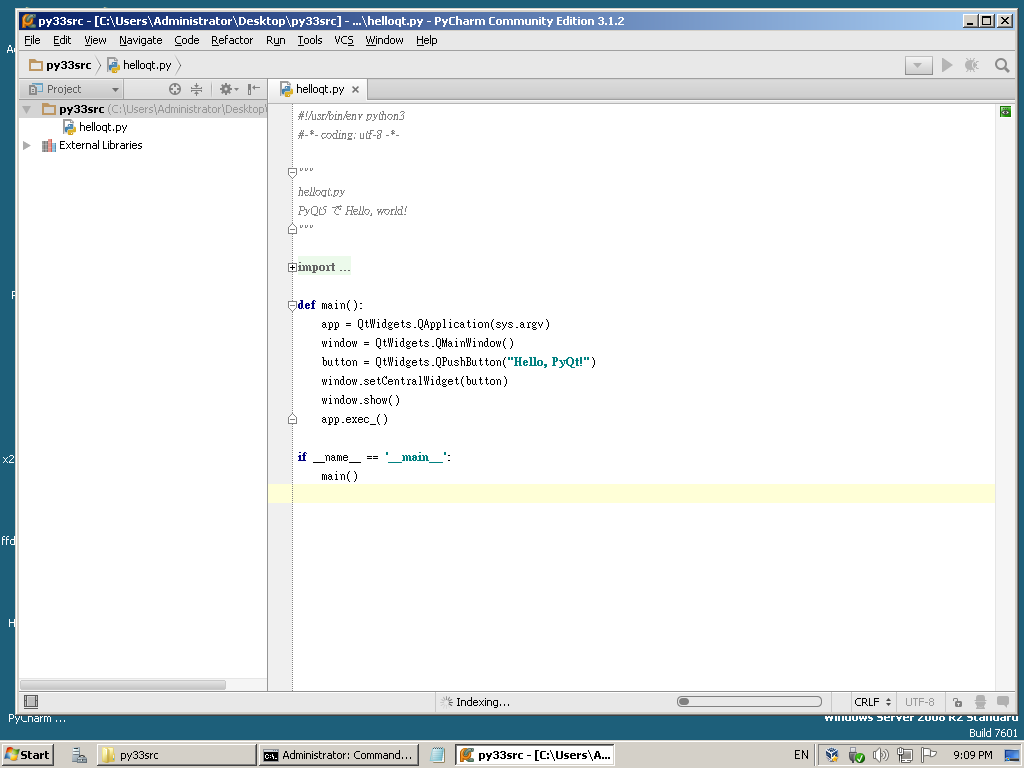
to run it
in the command prompt, change directory to your python.exe path, 'cd \python33' (if you are not in that path), type ' python.exe c:\Users\Administrator\Desktop\py33src\helloqt.py ', you will get your hello world run
compile/pack/freeze python source code to exe for platform without python,
in command prompt, change directory to C:\Python33\Scripts by typing ' cd c:\python33\scripts '
type and run:
cxfreeze.bat --base-name=Win32GUI c:\Users\Administrator\Desktop\py33src\helloqt.py
you will get helloqt.exe and its dependence files inside C:\Python33\Scripts\dist
note that --base-name=Win32GUI here is to hide the console window, you can try to compile without it to test the result#!/usr/bin/env python3
#-*- coding: utf-8 -*-
"""
helloqt.py
PyQt5 で Hello, world!
"""
import sys
from PyQt5 import QtWidgets
def main():
app = QtWidgets.QApplication(sys.argv)
window = QtWidgets.QMainWindow()
button = QtWidgets.QPushButton("Hello, PyQt!")
window.setCentralWidget(button)
window.show()
app.exec_()
if __name__ == '__main__':
main()
copy and paste the code to your IDE, for example, PyCharm, at this moment you can use windows notepad and save the file to (for example): C:\Users\Administrator\Desktop\py33src\helloqt.py
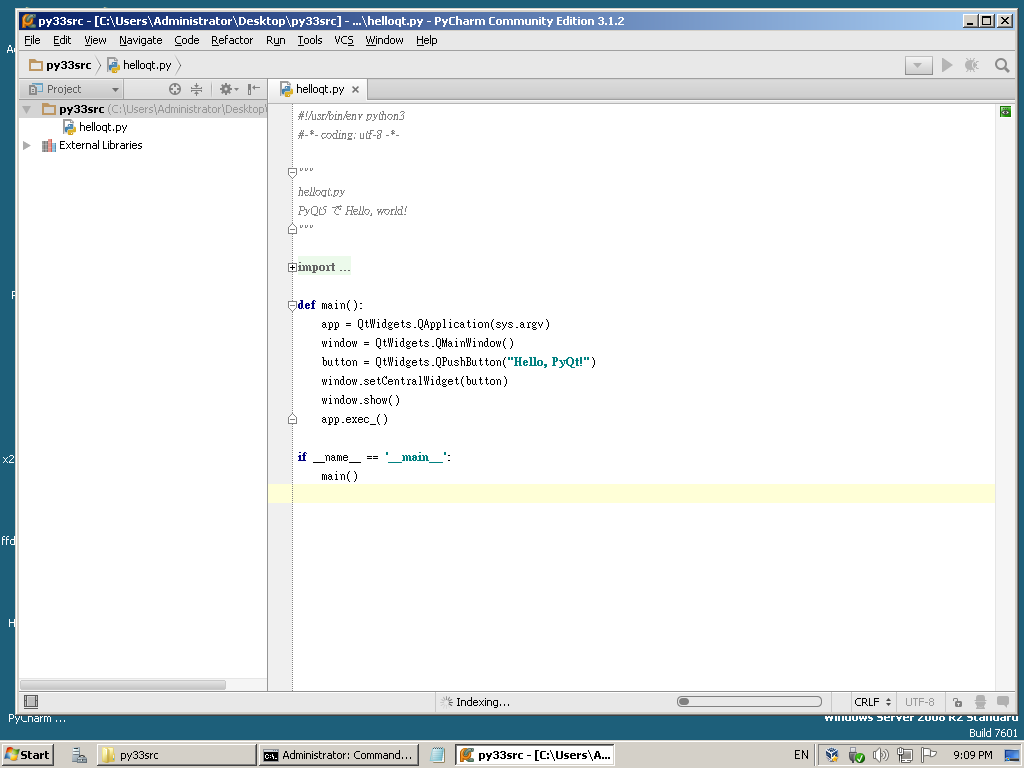
to run it
in the command prompt, change directory to your python.exe path, 'cd \python33' (if you are not in that path), type ' python.exe c:\Users\Administrator\Desktop\py33src\helloqt.py ', you will get your hello world run
compile/pack/freeze python source code to exe for platform without python,
in command prompt, change directory to C:\Python33\Scripts by typing ' cd c:\python33\scripts '
type and run:
cxfreeze.bat --base-name=Win32GUI c:\Users\Administrator\Desktop\py33src\helloqt.py
you will get helloqt.exe and its dependence files inside C:\Python33\Scripts\dist
remind that libEGL.dll should be copied from C:\Python33\Lib\site-packages\PyQt5 to the same folder of the outputted .exe for the machine with no PyQt5 installed, otherwise the program will complain 'This application failed to start because it could not find or load the Qt platform plugin "windows"'
https://bitbucket.org/anthony_tuininga/cx_freeze/issue/51/pyqt5-python33-cx_freeze432
http://stackoverflow.com/questions/20495620/qt-5-1-1-application-failed-to-start-because-platform-plugin-windows-is-missi
PyQt5 Reference Guide:
http://pyqt.sourceforge.net/Docs/PyQt5/
http://www.thehackeruniversity.com/2014/01/23/pyqt5-beginner-tutorial/
Download JetBrains Python IDE :: PyCharm:
http://www.jetbrains.com/pycharm/download/
Download JetBrains Python IDE :: PyCharm:
http://www.jetbrains.com/pycharm/download/